Cross Platform File Locking with Go
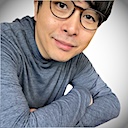
Founder and CEO
File locking controls concurrent access to files, preventing data corruption and inconsistent reads. The term is often associated with operating system file locks, but applications can also implement their own locking mechanisms. This post explores how to implement cross-platform operating system level file locking with Go.
Developing cross-platform applications can be tricky, particularly when dealing with OS-specific features like file locking. While Go’s standard library does not provide a public cross-platform API for file locking, Go provides build constraints (also known as build tags) as a conditional compilation feature. Build constraints can control which code is compiled for different target operating systems, ensuring implementations use the appropriate system calls for each platform. They determine which source files to include during compilation, allowing software developers to maintain platform-specific implementations in separate files. The following examples demonstrate how this works:
lock_unix.go
// go:build linux || darwin
import "syscall"
func Lock(f *os.File) error {
return syscall.Flock( ... )
}
func Unlock(f *os.File) error {
return syscall.Flock( ... )
}
lock_windows.go
// go:build windows
import "golang.org/x/sys/windows"
func Lock(f *os.File) error {
return windows.LockFileEx( ... )
}
func Unlock(f *os.File) error {
return windows.UnlockFileEx( ... )
}
During compilation, Go will only include the appropriate implementation file for the target operating system. Build constraints are not limited to just operating systems. They can also control compilation based on architecture, product tiers, or custom business requirements, making them a versatile tool for managing code variants.
In summary, Go’s build constraints can abstract away the complexity of platform dependent system calls behind a simple Lock()
/Unlock()
interface.
This pattern extends beyond operating systems, providing a clean approach for any functionality that requires platform-specific, architecture-dependent, or product tier implementations.